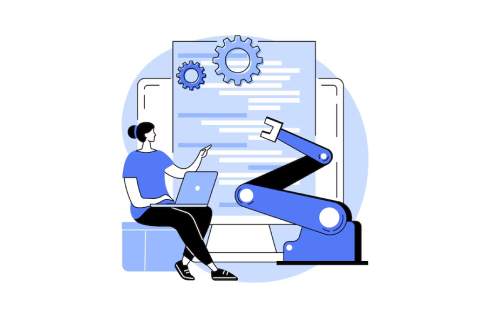
Automating the generation and management of a Software Bill of Materials (SBOM) is essential for software transparency and software supply chain security. This short guide introduces a sample Bash script that simplifies the process by generating an SBOM using cdxgen and uploading it to OWASP Dependency-Track for continuous monitoring.
How It Works
We’ll reference the code attached at the end of this document.
The script combines SBOM generation and seamless integration with Dependency-Track’s API. We would recommend that this code is incorporated into your CI/CD pipeline to ensure your software components are monitored for vulnerabilities and license compliance following all code changes. Here’s an overview of the key steps within the attached code script:
1. Check for cdxgen Installation (STEP 1)
The script first ensures that cdxgen, the tool used for SBOM generation, is installed. If not, it halts execution with an error message.
To download and install cdxgen, please refer to website: https://github.com/CycloneDX/cdxgen
2. Generate the SBOM (STEP 2)
The script navigates to your project’s directory, then uses cdxgen to create an SBOM in JSON using the CycloneDX format. This SBOM contains all the project’s dependencies and metadata and is in the appropriate format for ingestion into Dependency-Track.
3. Validate the SBOM (STEP 3)
Before proceeding, the script verifies that the SBOM file exists to avoid uploading invalid or incomplete data.
4. Upload to Dependency-Track (STEP 4)
The script uses curl to send the generated SBOM to Dependency-Track via its REST API. It includes project metadata (e.g., project name, version) to ensure proper tracking.
5. Error Handling (STEP 5)
If the upload fails, the script logs the HTTP status code and response for debugging purposes. A success message is displayed if the upload completes without issues.
Script Variables
The script uses customizable variables to adapt to different project environments:
● PROJECT_DIR: Directory of the project.
● PROJECT_NAME and PROJECT_VERSION: Name and version of the project for Dependency-Track tracking.
● PARENT_NAME and PARENT_VERSION: Optional metadata for parent projects.
● DT_URL: Dependency-Track API endpoint.
● API_KEY: API key for authenticating with Dependency-Track.
● SBOM_FILE: Name of the SBOM file generated by cdxgen.
How to Use the Script
1. Customize Variables:
○ Replace placeholders (e.g., your_project_directory, xxxx) with your project details and Dependency-Track configuration.
2. Make the Script Executable:
3. chmod +x generate_and_upload_sbom.sh
4. Run the Script:
5. ./generate_and_upload_sbom.sh
Appendix: CODE SCRIPT REFERENCED
#!/bin/bash
# Exit on any error
set -e
# Variables (Customize these)
PROJECT_DIR="your_project_directory"
PROJECT_NAME="xxxx"
PROJECT_VERSION="xxxx.SNAPSHOT"
PARENT_NAME="xxxx"
PARENT_VERSION="xxxx"
DT_URL="http://dtrack.example.com/api/v1/bom"
API_KEY="xxxxxx"
SBOM_FILE="sbom.json" # Adjust if using XML output
# Ensure cdxgen is installed (STEP 1)
if ! command -v cdxgen &> /dev/null; then
echo "cdxgen is not installed. Please install it and try again."
exit 1
fi
# Navigate to the project directory
cd "$PROJECT_DIR" || { echo "Directory $PROJECT_DIR does not exist."; exit 1; }
# Generate SBOM (STEP 2)
echo "Generating SBOM using cdxgen..."
cdxgen -o "$SBOM_FILE"
echo "SBOM generated: $SBOM_FILE"
# Validate SBOM file exists (STEP 3)
if [[ ! -f "$SBOM_FILE" ]]; then
echo "SBOM file $SBOM_FILE not found. Exiting."
exit 1
fi
# Upload SBOM to Dependency-Track (STEP 4)
echo "Uploading SBOM to Dependency-Track..."
response=$(curl -X "POST" "$DT_URL" \
-H 'Content-Type: multipart/form-data' \
-H "X-Api-Key: $API_KEY" \
-F "autoCreate=true" \
-F "projectName=$PROJECT_NAME" \
-F "projectVersion=$PROJECT_VERSION" \
-F "parentName=$PARENT_NAME" \
-F "parentVersion=$PARENT_VERSION" \
-F "bom=@$SBOM_FILE" \
-s -w "%{http_code}")
# Extract HTTP status code (STEP 5)
http_code="${response: -3}"
if [[ $http_code -eq 200 || $http_code -eq 201 ]]; then
echo "SBOM successfully uploaded to Dependency-Track."
else
echo "Failed to upload SBOM. HTTP Status: $http_code"
echo "Response: $response"
exit 1
fi
In conclusion, automating SBOM creation and analysis with tools like cdxgen and OWASP Dependency-Track is a powerful way to enhance software supply chain security. This simple yet effective Bash script allows seamless integration into your CI/CD pipelines, ensuring continuous monitoring for vulnerabilities and license compliance. By adopting this approach, you not only streamline your processes but also reinforce transparency and risk management across your software ecosystem. Start leveraging automation today to stay ahead in securing your applications.